As the world becomes ever more diverse, it is becoming increasingly common for IT pros to work with people who have a native language that is different from their own. Language barriers can present any number of challenges, but an inability to share PowerShell scripts with someone who speaks a different language doesn’t have to be one of them. It’s relatively easy to write multilingual PowerShell scripts. In this article, I will show you how it’s done.
Multilingual PowerShell scripts: Get-Culture to the rescue
The first step in building a multilingual PowerShell script is to figure out what language the person who is running the script prefers to use. You could write code that asks the person to select their preferred language, but a better approach may be to write the script to use the same language that the operating system is using. PowerShell includes a cmdlet called Get-Culture that can help with this. Check out these three lines of code:
$Culture = Get-Culture $Language = $Culture.Name $Language
The Get-Culture cmdlet returns a number of attributes related to the localization that the operating system is using. These include the user’s language, keyboard layout, date and time format, and more. The $Culture = Get-Culture command maps all of these attributes to a variable named $Culture. Since we are interested in language specifically, I created another variable called $Language that maps to the $Culture variable’s Name attribute. The $Language command outputs the $Language variable’s contents. As you can see in the screenshot below, the value that is returned is en-US (American English).
So, now that we have managed to retrieve the language that the operating system is using, the next step is to write a script that generates language specific output. In the interest of keeping things as simple as I can, I am going to be creating a variation of the old Hello World script.
For those who might not be familiar with Hello World, it is the name of the first program that most computer programming students ever write. The only thing that it does is to display the words Hello World on the screen. In PowerShell, we can do this with a single line of code:
Write-Host ‘Hello World’
But what if we wanted to create a multilingual Hello World script? One way of doing this would be to create a series of If Then statements that compare the contents of the $Language variable against a predetermined value and then output text based on the language indicated by the variable. Even though this approach would work, however, it gets a little bit messy. It probably wouldn’t be a big deal to write a Hello World script in this way, but just imagine if you were building a PowerShell script that contained numerous commands that output text.
Before I show you a slightly more elegant solution to this problem, there is one more piece of the puzzle that I need to talk about. As I have already indicated, EN-US is American English, but you will need to know the abbreviations for any other languages that you intend to support. Thankfully, Microsoft provides a list of these abbreviations here.
For the sake of demonstration, I am going to write my multilingual Hello World script to support three languages. I will be using American English (EN-US), Mexican Spanish (ES-MX), and Canadian French (FR-CA). So with that said, here are the phrases that I want the multilingual script to output based on the language that has been detected:
- American English: Hello World
- Mexican Spanish: Hola Mundo
- Canadian French: Bonjour le Monde
As I mentioned before, it would be possible to accomplish the task at hand by using If Then statements, but that approach gets a little bit messy if you have a lot of output to generate. A better approach is to use a switch statement. A switch statement works similarly to a collection of If Then statements, but without requiring you to write a gazillion If Thens. Instead, the If Then commands are more or less implied. So let’s take a look at how such a script might work. Here is the script:
$Culture = Get-Culture $Language = $Culture.Name Switch ($Language) { ‘EN-US’ {$LocalizedOutput = ‘Hello World’} ‘ES-MX’ {$LocalizedOutput = ‘Hola Mundo’} ‘FR-CA’ {$LocalizedOutput = ‘Bonjour le Monde’} } $LocalizedOutput
As you can see, this script starts by initializing the $Culture and $Language variables as described earlier so that $Language contains the language that is being used. Next, I created a Switch statement. Notice that the Switch command references the $Language variable. This means that the $Language variable’s contents will be used as the basis for comparison.
The next three lines of code work similarly to If Then statements. The first part of each of the three lines includes a value (EN-US, ES-MX, and FR-CA). As PowerShell processes this portion of the code, it will compare these values against the contents of the $Language variable. If it finds a match, then it will do whatever command is listed to the right of the value. In this case, I am creating a variable named $LocalizedOutput and populating the variable with a string of text that corresponds to the language that is being used. I could have included Write-Host commands instead, but I opted to simply map the output text to a variable because this approach makes it easier to extend the concept to more advanced scripts, such as those that rely on functions.
The last line in the script simply outputs the text that has been assigned to the $LocalizedOutput variable. You can see my script and its output in the figure below.
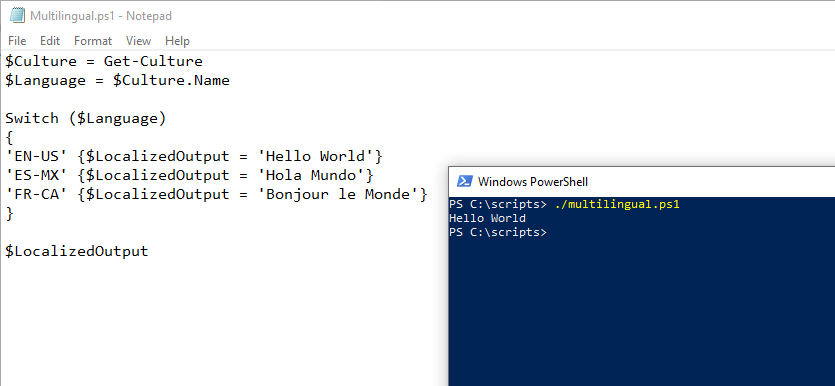
So useful for multinational companies
While not every organization currently requires multilingual PowerShell scripts, such scripts will almost certainly be useful to organizations that have IT resources located around the world.
Featured image: Shutterstock