Hash tables are easily one of the most useful data structures that you can create in PowerShell. A hash table is essentially a list of key/value pairs. These tables have countless uses. I often use hash tables as data lookup tables to query the hash table based on its key and have the corresponding value returned. In this article, I will show you how hash tables work.
Assigning a variable to an empty hash table
Creating an empty hash table is a really easy process. All you have to do is assign a variable to the empty hash. If, for example, you wanted to create a hash table named $HashTable, you could do so by using the following command:
$HashTable = @{}
You might have noticed that this command looks a lot like the command used to create an array. The difference is that if you wanted to create an array, you would use parenthesis, whereas if you want to create a hash table, you would use braces. Here is a comparison of the two:
$HashTable = @{} $SampleArray = @()
Once you have created a hash table, the next thing that you will need to do is to add some data to it. You can manually add data to a hash table, but in the real world, data is more commonly added by using a looping structure within a script.
Regardless of which method you use to add data to the hash table, you will need to add the data as a key/value pair. This key/value pair will serve as an associative lookup table. Let’s create a hash table containing a list of U.S. states and their capitals to show you how this works. Since adding all 50 states is probably overkill, I will demonstrate the concept with a few random states. Here is how it works:
$Capitals = @{} $Capitals += @{SC = ‘Columbia’} $Capitals += @{CA = ‘Sacramento’} $Capitals += @{KY = ‘Louisville’} $Capitals += @{MA = ‘Boston’}
Once you have created the hash table, you can view its contents the same way you view any other variable. Just enter the hash table’s name. In this case, you would type $Capitals. You can see an example of all of this in the image below.
I’m sure that some of you noticed that this table listed Louisville as the capital of Kentucky, whereas the capital is actually Frankfort. I intentionally introduced that mistake because I wanted to show you how to change a value stored within a hash table.
Changing a value
To change a value within a hash table, you will have to append .GetEnumerator() to your hash table variable. This will then allow you to look up a known value. In this case, that value is Louisville. Since our goal is to replace Louisville with Frankfort, we would need to append a pipe symbol, a percentage sign, and then assign a replacement value. Here is what the command looks like:
@($Capitals.GetEnumerator()) | Where-Object {$_.Value -eq ‘Louisville’} | % { $Capitals[$_.Key] = ‘Frankfort’}
You can see how this command works in the following image. I have entered the command shown above and then outputted the hash table’s contents to confirm that the value has indeed been changed.
I wanted to show you this particular method of updating a hash table because this is the way that I have always done it. However, there is a much easier way to change a value within a hash table. This method involves appending .Set_Item to the end of the hash table’s name. From there, you can simply provide the name of the key that needs to be changed along with its new value. In this case, the key would be KY and the value would be Frankfort. Here is what the command looks like:
$Capitals.Set_Item(“KY”,”Frankfort”)
You can see how this command works in the screenshot below.
As you can see, this is a much easier way of updating a value within a hash table.
At the beginning of this article, I mentioned that hash tables are often used as lookup tables within PowerShell scripts. That being the case, let’s see how you would look up a value using the hash table we have created.
The technique used to look up a value is actually very similar to the second technique that I showed you for updating a value. Rather than using Set_Item, however, you would use Get_Item. Similarly, there is no need to specify both the key and the value (especially since the goal is to look up the value). Instead, we need only to provide the key.
Imagine for a moment that you wanted to look up the capital of South Carolina using the hash table that we created earlier. The command used for doing so is:
$Capitals.Get_Item(“SC”)
You can see how this works in the screenshot below.
The command that I just showed you displays the requested value on the screen. If you are looking up a value from within a PowerShell script, you will typically take some sort of action based on the value. That being the case, you will typically need to map the value to a variable. To do so, you need only to append a variable assignment to the command that I just showed you. Here is what such a variable assignment might look like:
$SC_Capital = $Capitals.Get_Item(“SC”)
You can see how this process works in the next image.
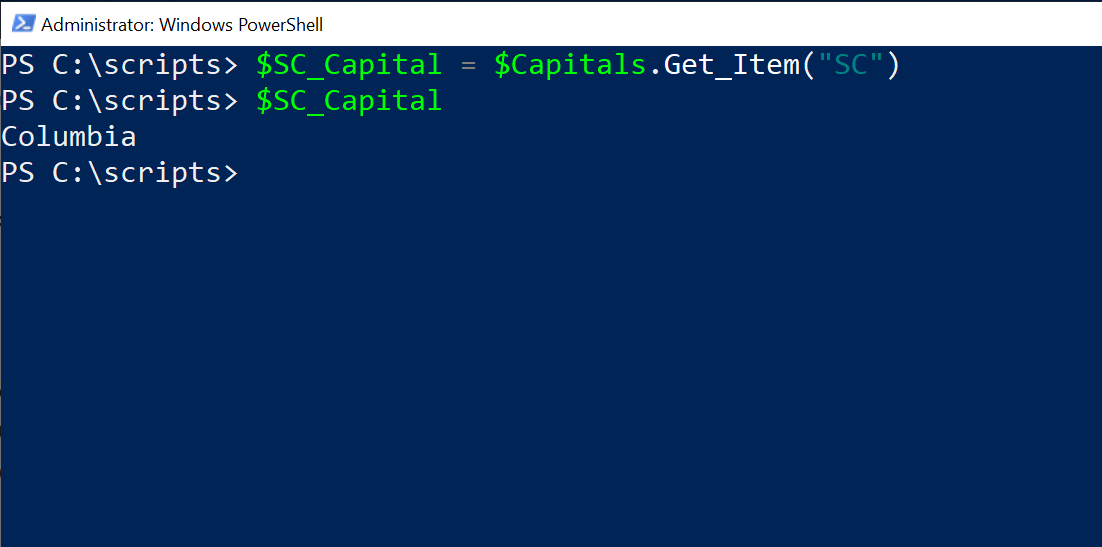
PowerShell hash tables: A useful tool in your toolbox
Hash tables can be extremely handy. They are one of the PowerShell data structures that I find myself using most often. You can even configure PowerShell to read data from a file and add that data to a hash table. In a separate article, for example, I talked about how to import and read data from a Windows INI file and map it to a PowerShell hash table.
Featured image: Shutterstock